二叉树(前、中、后序遍历)
class BinaryTree(object):
def __init__(self,data):
self.__left = None
self.__right = None
self.__data = data
def __del__(self):
del self.__data
def add_left_node(self,data):
if self.__left:
print("Left node exist!")
else:
self.__left = BinaryTree(data)
return self.__left
def add_right_node(self,data):
if self.__right:
print("Right node exist!")
else:
self.__right = BinaryTree(data)
return self.__right
def show(self):
print(self.__data)
def pre_order(self):#前序遍历
print(self.__data,end=" ")
if self.__left:
self.__left.pre_order()
if self.__right:
self.__right.pre_order()
def in_order(self):#中序遍历
if self.__left:
self.__left.in_order()
print(self.__data,end=" ")
if self.__right:
self.__right.in_order()
def post_order(self):#后序遍历
if self.__left:
self.__left.post_order()
if self.__right:
self.__right.post_order()
print(self.__data,end=" ")
if __name__ == "__main__":
root = BinaryTree("root")
a = root.add_left_node("A")
b = root.add_right_node("B")
c = a.add_right_node("C")
d = b.add_left_node("D")
e = b.add_right_node("E")
root.show()
root.pre_order()
print("")
root.in_order()
print("")
root.post_order()
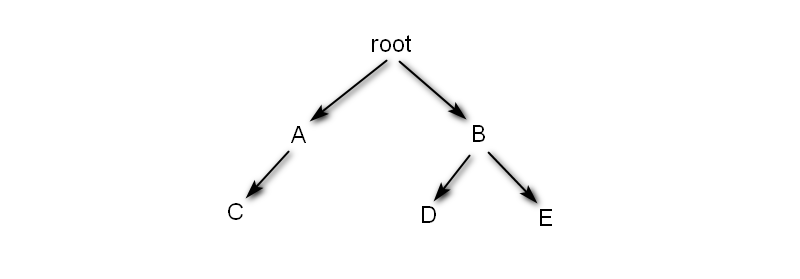
运行结果:
root
root A C B D E
A C root D B E
C A D E B root
小根堆
heapq 模块提供了堆算法。heapq是一种子节点和父节点排序的树形数据结构。这个模块提供heap[k] <= heap[2*k+1]
并且heap[k] <= heap[2*k+2]
,根的下标从0开始。
import heapq
import random
data = random.sample(range(101),10)#生成10个100以内的随机测试数据
print(data)
heapq.heapify(data)#堆化测试数据
print(data)
heapq.heappush(data,28)#新元素入堆,并重新堆化所有数据
print(data)
heapq.heappop(data)#删除最小数据,并重新堆化所有数据
print(data)
heapq.heappushpop(data,100)#删除最小元素,新元素入堆,并重新堆化所有数据
print(data)
heapq.heapreplace(data,50)#功能和heapq.heappushpop一样
print(data)
maxs = heapq.nlargest(3,data)
print(maxs)
mins = heapq.nsmallest(3,data)
#mins = heapq.nsmallest(3,data,key=str)按指定规则返回最小的3个元素
print(mins)
运行结果:
[80, 55, 34, 75, 15, 83, 56, 38, 70, 35]
[15, 35, 34, 38, 55, 83, 56, 75, 70, 80]
[15, 28, 34, 38, 35, 83, 56, 75, 70, 80, 55]
[28, 35, 34, 38, 55, 83, 56, 75, 70, 80]
[34, 35, 56, 38, 55, 83, 100, 75, 70, 80]
[35, 38, 56, 50, 55, 83, 100, 75, 70, 80]
[100, 83, 80]
[35, 38, 50]
凯撒移位
import string
low_case = string.ascii_lowercase
up_case = string.ascii_uppercase
before = low_case + up_case #abcdefghijklmnoqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ
print("======== 欢迎使用凯撒移位 ========")
print(" Author:Mochazz")
test_string = input('请输入你要解密的密文:')
for i in range(1,26):
after = low_case[i:] + low_case[:i] + up_case[i:] + up_case[:i]
# print(after)
table = ''.maketrans(before,after)
print('移%d位:'%i + test_string.translate(table))
运行结果:
======== 欢迎使用凯撒移位 ========
Author:Mochazz
请输入你要解密的密文:Uif rvjdl cspxo gpy kvnqt pwfs uif mbaz eph
移1位:Vjg swkem dtqyp hqz lworu qxgt vjg ncba fqi
移2位:Wkh txlfn eurzq ira mxpsv ryhu wkh odcb grj
移3位:Xli uymgo fvsar jsb nyqtw sziv xli pedc hsk
移4位:Ymj vznhp gwtbs ktc ozrux tajw ymj qfed itl
移5位:Znk waoiq hxuct lud pasvy ubkx znk rgfe jum
移6位:Aol xbpjr iyvdu mve qbtwz vcly aol shgf kvn
移7位:Bpm ycqks jzwev nwf rcuxa wdmz bpm tihg lwo
移8位:Cqn zdrlt kaxfw oxg sdvyb xena cqn ujih mxp
移9位:Dro aesmu lbygx pyh tewzc yfob dro vkji nyq
移10位:Esp bftnv mczhy qzi ufxad zgpc esp wlkj ozr
移11位:Ftq cguow ndaiz raj vgybe ahqd ftq xmlk pas
移12位:Gur dhvpx oebja sbk whzcf bire gur ynml qbt
移13位:Hvs eiwqy pfckb tcl xiadg cjsf hvs zonm rcu
移14位:Iwt fjxrz qgdlc udm yjbeh dktg iwt apon sdv
移15位:Jxu gkysa rhemd ven zkcfi eluh jxu bqpo tew
移16位:Kyv hlztb sifne wfo aldgj fmvi kyv crqp ufx
移17位:Lzw imauc tjgof xgp bmehk gnwj lzw dsrq vgy
移18位:Max jnbvd ukhpg yhq cnfil hoxk max etsr whz
移19位:Nby kocwe vliqh zir dogjm ipyl nby futs xia
移20位:Ocz lpdxf wmjri ajs ephkn jqzm ocz gvut yjb
移21位:Pda mqeyg xnksj bkt fqilo kran pda hwvu zkc
移22位:Qeb nrfzh yoltk clu grjmp lsbo qeb ixwv ald
移23位:Rfc osgai zpmul dmv hsknq mtcp rfc jyxw bme
移24位:Sgd pthbj aqnvm enw itlor nudq sgd kzyx cnf
移25位:The quick brown fox jumps over the lazy dog
类型转换
类型转换可以使用str()、list()、dict()等内置方法,当需要对大量数据进行类型转换时,内置函数map()可以提供非常高的效率。
import timeit
t1 = timeit.timeit('"=".join([str(n) for n in range(100)])',number=10000)
print('耗时:',t1)
t2 = timeit.timeit('"=".join(str(n) for n in range(100))',number=10000)
print('耗时:',t2)
t3 = timeit.timeit('"=".join(map(str,range(100)))',number=10000)
print('耗时:',t3)
运行结果:
耗时: 0.31382967308271886
耗时: 0.34856061037248814
耗时: 0.24415366054608445
字符串加解密
from itertools import cycle
def crypt(source,key):
result = ''
temp = cycle(key)#将key首尾相连形成一个圆环,使用next会一直循环
for ch in source:
result += chr(ord(ch)^ord(next(temp)))
return result
source = input('请输入你要加密的明文:')
key = input('秘钥为:')
encrypted = crypt(source,key)
print('加密后的密文为:%s' % encrypted)
print('解密后的密文为:%s' % crypt(encrypted,key))
运行结果:
请输入你要加密的明文:Mochazz
秘钥为:hack
加密后的密文为:%
解密后的密文为:Mochazz
加解密原理:
其实就是用了^(异或)运算
加密:string^key=明文^秘钥=密文
解密:string^key^key=密文^秘钥=string^0=string=明文
pycrypto
Python3.5不支持pycrypto,使用以下命令可以安装pycrypto
pip install --use-wheel --no-index --find-links=https://github.com/sfbahr/PyCrypto-Wheels/raw/master/pycrypto-2.6.1-cp35-none-win_amd64.whl pycrypto
使用rsa模块实现消息加解密
import rsa
key = rsa.newkeys(3000)
public_key = key[0]
private_key = key[1]
msg = "Mochazz"
print("Before:%s" % msg)
msg = msg.encode()
crypto_msg = rsa.encrypt(msg,public_key)
print("Crypto:%s" % crypto_msg)
decrypto_msg = rsa.decrypt(crypto_msg,private_key)
print("Decrypto:%s" % decrypto_msg.decode())
运行结果:
Before:Mochazz
Crypto:b'.\x1b\x05\xf6\x9a\xf4\x93\x0b-P+\xda\xcd\xa0\x05\xa11rxEl0F\xceZ\xa9\x814\xb8\x93\xcfu@?\xca\x06\xae\xcc3\xe3H\xde+\x84\xe8s\xbdL"&\x86\xf4\x165\x89\x8d\xd4\xcf\xd9\x89j\xf4\x91lX\x9f\xe4\xf29c\x97\xee\xc5w\x87\x06\xc0\x1b\x99\xa1\x9e\xf1\xdf\xb0\xd41?\x94|\x9a\xf9\x9f\xc7\xa5uz\x8e\xa8\x07\xfc\x89\xd5\t\\y\x0e\x91\x7f\xf7\x9cZ\xade\xf5.\x05\x8e)|\x1fp\x9f)[m\xd0\x18\xd6g,\x1a\x82Mc\x82\x1b|+\x97\x1c\x9e\x91\x01\x08\xf8V\xd2?\xf0(\xf8pf;\x05$Y\x14\x1d\x85h\x85\xde\xcd\xf2\xdetU\xb1(\xf2\x03x\x82\xcf\xc9$\x85\xd3\x08|=cF\x82z\x8f\x04%\x8a\x0f\xa0\xebB"=\xeb\xcf\xee\x1b\xa4\xefr+\xc8\xaau\xed~\xd7P\xe9\xbez]\xd5\xe1\x1cjBs;z`\xfbrZ6Xc\xb5\x80\xe9\x95\xd0tL\x84p\xf7\xf6,\xcd\xd9\xbb^g\xa0\x83\xeb\xad]\x8e\x9d\xf3\xc6F\xc9\xa07\r $\x89=\x19x^\'\x82\x18\xdf\xc9\'\x9aq\xba^\xab\xc4\xea\x06\x04)\xf7\x99A\x8e\x11\xcf\xd3\xb1\x9c\xffS\xa5I[n\xa1\xfa+\xedw\x1fF\xec\xe9\x0c\xff\x03D\x17\x9b:G\xea\x92W\xdd\xc7\xce\x8f\x85\x8c\x9c\xef\xb7\xf5H\xe9\x17\r-\xde\x1f\x00\xd9r\x9b\xaa\x11\xfb\xfa\x14i$\x02\xf0\x15?\xa0q\x98\xb6\x12\xd6\xe2s[S \x06S\x19\x92\xb4\x94L~3\xb9)\xf8\x9b'
Decrypto:Mochazz